The RUN
, ENTRYPOINT
, and CMD
, instructions all have two different forms they can be written in, and those forms change how each of those instructions behaves.
The two forms
Shell form:
Commands are written without
[]
brackets and are run by the container's shell, such as/bin/sh -c
. Example:FROM alpine:latest # /bin/sh -c 'echo $HOME' RUN echo $HOME # /bin/sh -c 'echo $PATH' CMD echo $PATH
Depending on the shell, commands will execute as child processes of the shell, which has some potentially negative consequences at the cost of some features, described below.
Exec form:
Commands are written with
[]
brackets and are run directly, not through a shell. Example:FROM alpine:latest RUN ["pwd"] CMD ["sleep", "1s"]
This removes a potentially extra process in the process tree, which may be desirable.
Recommended forms
These are the recommended forms to use for each instruction:
RUN
: shell form, because of the shell features described belowENTRYPOINT
: exec form, because of the signal trapping described belowCMD
: exec form, because of the signal trapping described below
The general idea is to use the exec form unless you need shell features - and if you need shell features in the ENTRYPOINT
or CMD
, then consider writing a shell script and executing it with the exec form.
Use cases
There are real use cases where you may not want to follow the above recommendations, so let's explore the main consequences of the two forms.
Variable substitution
In the shell form, commands will inherit environment variables from the shell, such as $HOME
and $PATH
:
FROM alpine:latest
# Shell: echoes "/root" as set by the shell
RUN echo $HOME
# Exec: echoes "$HOME" because nothing is set
RUN ["echo", "$HOME"]
However, both forms behave the same when it comes to environment variables set by the ENV
instruction:
FROM alpine:latest
ENV VERSION=1.0.0
# Shell: echoes "1.0.0" because Docker does the substitution
RUN echo $VERSION
# Exec: echoes "1.0.0" because Docker does the substitution
RUN ["echo", "$VERSION"]
Shell features
The main thing you lose with the exec form is all the useful shell features: sub commands, piping output, chaining commands, I/O redirection, and more. These kinds of commands are only possible with the shell form:
FROM ubuntu:latest
# Shell: run a speed test
RUN apt-get update \
&& apt-get install -y wget \
&& wget -O /dev/null http://speedtest.wdc01.softlayer.com/downloads/test10.zip \
&& rm -rf /var/lib/apt/lists/*
# Shell: output the default shell location
CMD which $(echo $0)
Most Dockerfiles are written with the shell form for RUN
for the niceties as well as layer reduction.
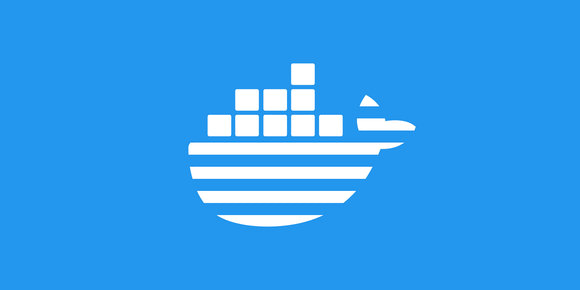
Reducing Docker Layers
Jun 29, 2020 · 5 min read
Reducing the size of your Docker images is important for a number of reasons, and while there are newer tools such as multi-stage builds, reducing the number of layers in your image may help.
Signal trapping & forwarding
Most shells do not forward process signals to child processes, which means the SIGINT
generated by pressing CTRL-C
may not stop a child process:
# Note: Alpine's `/bin/sh` is really BusyBox `ash`, and when
# `/bin/sh -c` is run it replace itself with the command rather
# than spawning a new process, unlike Ubuntu's `bash`, meaning
# Alpine doesn't exhibit the forwarding problem
FROM ubuntu:latest
# Shell: `bash` doesn't forward CTRL-C SIGINT to `top`
ENTRYPOINT top -b
# Exec: `top` traps CTRL-C SIGINT and stops
ENTRYPOINT ["top", "-b"]
This is the main reason to use the exec form for both ENTRYPOINT
and SHELL
.
CMD
as ENTRYPOINT
parameters
There's a special version of the exec form for CMD
where if the first item in the array isn't a command then all items will be used as parameters for the ENTRYPOINT
:
FROM alpine:latest
# Exec: default container command
ENTRYPOINT ["sleep"]
# Exec: not a command, so it becomes a parameter
CMD ["1s"]
This is not possible with the shell form.
Conclusion
In general, use the shell form for RUN
, and the exec form for everything else.